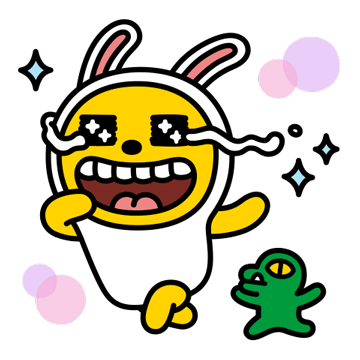
실제로 Data를 가지고 프로그램을 만들 때, 코드에 모든 것을 넣진 않는다.
대신 File을 Disk에 저장 후 프로그램이 이것을 읽거나 쓸 수 있도록 한다.
이번엔 Jupyternotebook이 아닌 Google Colab에서 코딩을 해봤당.
구글 Colab에 구글 Drive를 위와 같이 연결하고 Colab Notebooks 폴더에 Text파일을 집어 넣어서 테스트좀 해보려함
Opening a File
- 아래와 같이 외부파일을 읽어본다
- File을 Open했으면 끝에는 Close 해줄 것 --> Memory 쓸 데 없이 차지하는 것 방지용
file = open('textfile.txt','r')
contents = file.read()
file.close()
print(contents)
---------------------------
test text file
This is the second line!
- 같은 Directory에 있는 File을 읽어본 것
- file = open(<file_name>,<mode>) --> Mode : 'r' - reading / 'w' - writing / 'a' - appending
- file.read() : 전체 file을 읽어서 String으로 저장한다 --> text file이 아닌 file을 읽으면 어떻게든 text로 변환하여 출력하기에 파일이 깨져보이는 현상 발생
- Pattern : 1st 소스얻기 : open() , 2nd 소스로 뭐든 하기 : read() , 3rd 소스 제거 : close()
- File Close 자동으로 적용시킬 수 있는 법
with open('textfile.txt','r') as file:
contents = file.read() #close 안 해줘도 자연스럽게 close다. with 안에서만 Local로 존재
print(contents)
File Path
- 코드가 실행중인 Directory에 파일이 있으면 위와 같이 바로 파일 동작 OK
- 파일이 다른 Path에 있다면?
- 내가 지금 어디 위치에 있는지 헷갈린다면?
import os
os.getcwd()
--------------------------------
/content/drive/MyDrive/Colab Notebooks
- Directory 바꾸고 싶다면
os.chdir("/content/drive/MyDrive/Colab Notebooks")
Reading Contents in a File
- 파일을 일부만 읽기 : Character 10개만 읽어보자
with open('textfile.txt','r') as file:
contents = file.read(10)
print(contents)
---------------------
test text
# file.read를 해 줄때마다 file read를 하는 커서는 0에서부터 계속 뒤로 이동한다.
with open('textfile.txt','r') as file:
contents = file.read(1)
contents = file.read(1)
contents = file.read(1)
contents = file.read(1)
contents = file.read(1)
contents = file.read(1)
contents = file.read(1)
contents = file.read(1)
--------------------------------
x
- Line by Line 으로 파읽 읽기
# For문
with open('textfile.txt','r') as file:
for line in file:
print(line)
--------------------
test text file
This is the second line!
# While문
with open('textfile.txt','r') as file:
line = file.readline()
while line:
print(line)
line = file.readline()
----------------------------
test text file
This is the second line!
#Read lines
with open('textfile.txt','r') as file:
line = file.readlines()
print(line)
['test text file\n', 'This is the second line!']
Writing Files
- Write
with open('file.txt','w') as output_file:
output_file.write('내용\n')
- file.txt가 없다면 파일 만들고 Write / 있다면 기존 내용을 덮어쓰기 해서 Write --> 기존 내용 날라감;
- Append
with open('file.txt','a') as output_file:
output_file.write('내용\n')
- 기존에 있던 내용에 더해서 내용을 추가한다. --> 안전성면에서 좋음
- \n 라인 바꿔주는 건 넣자. 아니면 가로줄로 길어진다.
Split()
- Noise가 많이 낀 txt파일을 가공할 때 많이 쓰인다.
- 특정 value 기준으로 txt파일을 분해해서 List파일로 변환해준다. (STRING을 특정기준으로 분리해주는 Method?!)
txt = "아아,그것은,말이지,나의,보물이야!"
after = txt.split(',')
after
['아아', '그것은', '말이지', '나의', '보물이야!']
Real Scenario
- 이름,학번,학점 으로 구분된 txt를 가공하는 시나리오
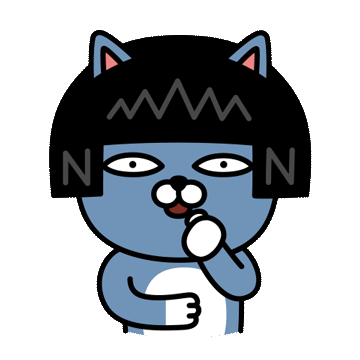
'SW 만학도 > Python' 카테고리의 다른 글
9. Computational Complexity & Searching (Big O with Search/Sort in Python) (2) | 2024.03.17 |
---|---|
8. Object-oriented Programming in Python (0) | 2024.03.15 |
6. Sets, Tuples, and Dictionaries (3) | 2024.03.15 |
5. Lists and Loops in Python (0) | 2024.03.14 |
4. Modules and Classes in Python (0) | 2024.03.13 |