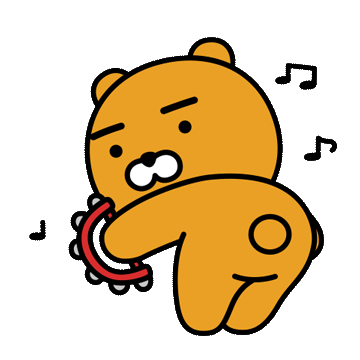
모듈과 클래스에 관한 설명이다.
이 또한 기본적인 것이니 심도 있게 학습해보자~
Module
- 모듈이란 Reusalbe한 Variables와 Functions 를 모아두는 것
- 어떤 모듈은 하나의 프로그램에서만 쓰이는 것이 아니라 다양한 곳에서 쓰인다.
- ex) import math --> math 안에 있는 function / variable을 쓸 수 있게 된다.
import math
print(math.sqrt(9))
print(math.pi)
3.0
3.141592653589793
- import 하는 모듈이름이 너무 길다면 as로 줄일 수 있다.
- ex) import numpy as np
- Module에서의 variable 값 / 함수 이름은 절대 바꾸지 말자! 코드가 꼬일 수 있다.
- ex) math.pi = 1 , math.max = math.min
- Module에서 특정한 func , var만 가져오는 법?
- from math import sqrt,pi
from math import pi,sqrt
print(pi,sqrt(16.0))
3.141592653589793 4.0
- 이렇게 func / var 하나만 가져와도 Memory 절약효과는 없다. 일단 모든 모듈을 메모리에 load를 하긴 해서용;
- 그래도 Typing이 간결해지니 그냥 사용하자~
- What if? : from math import * -> Typing 간결해지니 편하다! 그러나 비추.. Module 들을 이런 식으로 불러오다보면 Module끼리 이름이 같은 func , var이 있어서 코드가 꼬일 수 있다.
--> C에선 요런 것들을 error로 잡아주는데, 우리 free한 파이썬은 이런 것을 error로 잡지 않는다.
--> 즉, 난중에 이로 인해 error 발생 시 debugging이 매우 힘들 수도 있다는..
Making Own Module
- 내가 만든 Module을 py파일로 만든다.
- 같은 폴더에서 py파일을 만들어 위 Module을 사용해본다.(주피터노트북 사용시 download as py 파일로 저장)
import test1
print(test1.test2(5))
print(test1.test3(5))
6
13
Classes
클라스 있는 클래스는 무엇일까
추후 나올 Object-Oriented Programming에 필요한 타입!
- Class is a customized data type to represent a same kind of objects
- ex) 사람, 자동차, 나라.. 등 하나의 개념 또는 객체를 형상화한 것이 Class
- What's in Class?
1. Attributes : 객체의 특징을 나타내는 변수
2. Methods : 위 객체와 관련된 기능을 제공하는 함수
- ex) Class Person , Attribute : age, gender , Methods : change_gender() , age_recalculate()
- Class는 BLUEPRINT 청사진이다 vs Class Object는 class 내에 실제 존재하는 객체들(Instance of Class)
- ex)
-현대차 제네시스 설계도 : Class
-G90 1대 : Class Object
- Attribute : color, size ...
- Method : add_oil(), sell_car() ...
Built-In Class
- int class, float class, bool class, str class
- ex) str class
- Class object : job = 'student'
- method : job.lower() , job.find('u'), job.count('t') ...
- ex) 다양한 str class method
str1 = 'im a boy you are a girl love ya every body'
print(str1.count('a'))
print(str1.upper())
print(str1.find('a'))
print(str1.find('z'))
print(str1.lstrip('m'))
print(str1.lstrip('i'))
print(str1.rstrip('y'))
print(str1.split(' '))
print(str1.split())
4
IM A BOY YOU ARE A GIRL LOVE YA EVERY BODY
3
-1
im a boy you are a girl love ya every body
m a boy you are a girl love ya every body
im a boy you are a girl love ya every bod
['im', 'a', 'boy', 'you', 'are', 'a', 'girl', 'love', 'ya', 'every', 'body']
['im', 'a', 'boy', 'you', 'are', 'a', 'girl', 'love', 'ya', 'every', 'body']
안뇽~
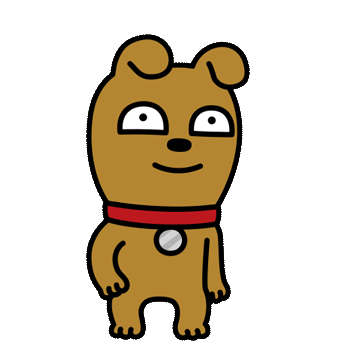
'SW 만학도 > Python' 카테고리의 다른 글
6. Sets, Tuples, and Dictionaries (2) | 2024.03.15 |
---|---|
5. Lists and Loops in Python (0) | 2024.03.14 |
3. String and Control in Python (0) | 2024.03.13 |
2. Python Functions (2) | 2024.03.12 |
1. Hello Python (1) | 2024.03.12 |