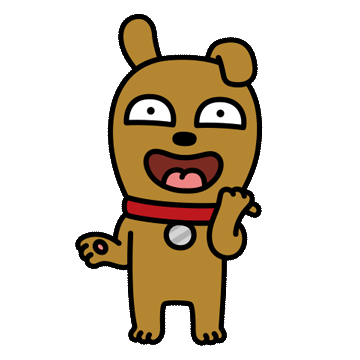
Python의 보다 다양한 자료구조를 알아보자.
다~ 나중에 요긴하게 쓰일 자료구조라 정확히 구분짓고 넘어가는 것이 중요!
Sets
- 집합
- 순서가 따로 없고, 모든 원소는 서로 달라야한다. Unordered and Distinct
- 원소를 추가/삭제할 순 있지만 수정할 순 없다. Immutable
alphabet = {'a','b','b','a','d','c','c','e'}
alphabet
{'a', 'b', 'c', 'd', 'e'}
- 중괄호롤 사용해 위와 같이 Set을 선언할 수 있다.
- 중복되는 것 제거 + 순서 마음대로 재정렬되는 것 확인(Unordered and Distinct)
#An empty set
empty = set()
empty1 = {} # 이것은 Dictionary!!
- 공집합은 set() 으로 만들어 준다.
- set 안에 List 넣어서 set 만들어주기 가능 --> set([1,3,2,2,5]) --> {1,2,3,5}
Methods with Sets
test1 = set([0,1,3,3,2,4,5,6,7,8])
test2 = {0,1,2,3}
print('test1=',test1,',test2=',test2)
test1.add(10)
print(test1)
test1.remove(10)
print(test1)
print(test1.issubset(test2)) #부분집합 여부
print(test1.issuperset(test2))
print(test1.difference(test2)) #차집합
print(test1.intersection(test2)) #교집합
print(test1.union(test2)) #합집합
----------------------------------------
test1= {0, 1, 2, 3, 4, 5, 6, 7, 8} ,test2= {0, 1, 2, 3}
{0, 1, 2, 3, 4, 5, 6, 7, 8, 10}
{0, 1, 2, 3, 4, 5, 6, 7, 8}
False
True
{4, 5, 6, 7, 8}
{0, 1, 2, 3}
{0, 1, 2, 3, 4, 5, 6, 7, 8}
Set is immutable
- Set은 내제된 Data check를 Hashing 이라는 스킬을 통해 굉장히 빨리 하는데, 이 때문에 Mutable한 원소를 갖지 못 한다. 그래서 List와 같이 Mutable한 원소를 Set에 추가하려고 하면 Error!
Tuples
- 튜플은 순서가 상관이 있고 원소를 바꿀 수 없다. Orderd and Immutable
- 튜플은 데이터 중복이 허용된다! Not Ditinct
- 튜플은 아래와 같이 Declare한다. 유의점은 원소가 하나 일 땐 쉼표를 써준다. 쉼표를 안 쓰면 튜플로 인식 안 하고 그냥 수학식 숫자로 간주
tuples = ()
print(tuples)
tuples = (4)
print(tuples)
tuples = (4,)
print(tuples)
tuples = (1+3)
print(tuples)
tuples = ('억')
print(tuples)
tuples = (1+3,)
print(tuples)
tuples = (1,3,3,2,[1,2,3])
print(tuples)
()
4
(4,)
4
억
(4,)
(1, 3, 3, 2, [1, 2, 3])
- 튜플의 경우 List를 원소로 받을 수 있고, 이 때 튜플 안에 있는 List의 값은 바꿀 수 있다.(List 주소는 고정! 다만 그 안에 있는 값은 바꿀 수 있다는 것)
student=(['Kim','A'],['Park','C'],100)
student[0]='Change'
---------------------------------------------------------------------------
TypeError Traceback (most recent call last)
Cell In[32], line 1
----> 1 student[0]='Change'
TypeError: 'tuple' object does not support item assignment
---------------------------------------------------------------------------
student[0]
['Kim', 'A']
student[0][0]='Lee'
student
(['Lee', 'A'], ['Park', 'C'], 100)
Dictionaries
dict_empty={}
dict_mem = {'Lee':'A','Park':'B','Park':'C'} # Lee / Park -> Keys , 'A','B','C' -> Valeus 키/벨류 둘이 합쳐서 Items
print(dict_mem)
print(dict_mem['Park'])
{'Lee': 'A', 'Park': 'C'}
C
- Update / Modify 가능! Mutable!
dict_mem = {'Lee':'A','Park':'B','Park':'C'}
dict_mem['Kim'] = 'Z'
print(dict_mem)
dict_mem['Kim'] = 'Y'
print(dict_mem)
print('Han' in dict_mem)
print('Kim' in dict_mem)
del dict_mem['Kim']
print(dict_mem)
-------------------------------------------
{'Lee': 'A', 'Park': 'C', 'Kim': 'Z'}
{'Lee': 'A', 'Park': 'C', 'Kim': 'Y'}
False
True
{'Lee': 'A', 'Park': 'C'}
- For loop with Dict
print(dict_mem)
for key in dict_mem:
print(key)
for x in dict_mem:
print([x,dict_mem[x]])
for key,value in dict_mem.items():
print(key,value)
-------------------------------------------
{'Lee': 'A', 'Park': 'C', 'Kim': 'Z'}
Lee
Park
Kim
['Lee', 'A']
['Park', 'C']
['Kim', 'Z']
Lee A
Park C
Kim Z
Methods with Dictionaries
- Dictionary ex)
- Key / Value를 뒤바꾼 Dictionary를 만들어 보자
dict_mem
{'Lee': 'A', 'Kim': 'Z', 'Park': 'C', 'Ahn': 'Z'}
dict_new={}
for key,value in dict_mem.items():
dict_new[value]=key #value가 'Z'으로 중복인 경우는 Key로 전환될 때 날라갈 수밖에 없다..
dict_new
{'A': 'Lee', 'Z': 'Ahn', 'C': 'Park'}
- Value가 중복이 있는 경우를 따져서 다시 코딩을 해보자
dict_mem={'Lee': 'A', 'Kim': 'Z', 'Park': 'C', 'Ahn': 'Z'}
dict_new={}
for key,value in dict_mem.items():
if value not in dict_new:
dict_new[value]=key
else:
dict_new[value]=[dict_new[value],key]
dict_new
{'A': 'Lee', 'Z': ['Kim', 'Ahn'], 'C': 'Park'}
# 이런 식으로 아예 새로운 value들을 전부 List로 지정해도 된다
dict_mem={'Lee': 'A', 'Kim': 'Z', 'Park': 'C', 'Ahn': 'Z'}
dict_new={}
for key, value in dict_mem.items():
if value in dict_new:
dict_new[value].append(key)
else:
dict_new[value]=[key]
dict_new
{'A': ['Lee'], 'Z': ['Kim', 'Ahn'], 'C': ['Park']}
Mutability Summary
자료구조 | Mutability | Ordered | 비고 |
String | X | O | Keep track of a text |
List | O | O | Keep track of an ordered sequence |
Tuple | X | O | Build an ordered seq that won't be change Value가 List이면 List 안 값은 바꿀 수 있다. |
Set | O (add / remove로 value 수정) |
X | Keep track of values. But order doesn't matter. And values must be immutable List 같은 Mutable 값 자체를 Value로 가지지 못한다. |
Dictionary | O | O | Mapping, Keys are immutable |
복잡한 자료구조의 세계..
코딩하다보면 자연스럽게 위 표는 손에 익혀진다고 한다..
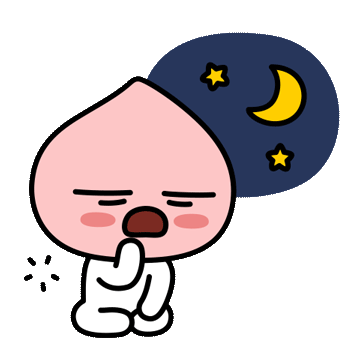
'SW 만학도 > Python' 카테고리의 다른 글
8. Object-oriented Programming in Python (0) | 2024.03.15 |
---|---|
7. File I/O in Python (1) | 2024.03.15 |
5. Lists and Loops in Python (0) | 2024.03.14 |
4. Modules and Classes in Python (0) | 2024.03.13 |
3. String and Control in Python (0) | 2024.03.13 |