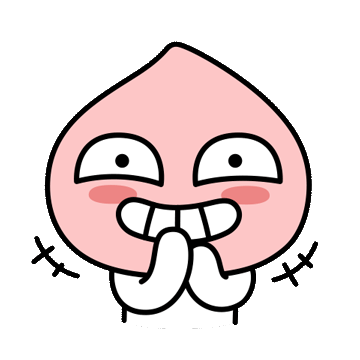
Python에서 굉장히 중요한 개념! 리스트와 루프!
Python으로 대량의 Data를 처리하려 할 때, 일일히 Variable을 설정하려면 너무 많다.
그래서 보통 사용하는게 List! 주요 자료구조 中 하나
- A1 = 'Mina' , A2 = 'Lisa' , A3 = 'Marcus' .... (X)
- People = ['Mina', 'Lisa', 'Marcus' ... ] (O) --> LIST!
Lists
- List는 포함하는 Item의 순서를 따진다! Ordered! 참고로 숫자는 0부터 센다 in python, C ...
- Why 0부터? --> 컴퓨터는 2진수를 쓰고, 2진수에서 가장 작은 수는 0이니까 0부터
Lists : Access and Assign
- ex)
- people = ['Jia Kang', 'Jihye Kang' ... , 'Sooyeon Hwang']
- Acess?
people[0] = 'Jia Kang' , people[1] = 'Jihye Kang' , people[-1] = 'Sooyeon Hwang'
- 따른 Variable에 Assign도 가능
ex) me = people[0] , me --> 'Jia Kang'
- Modify? 가능!
ex) people[0] = 'Marcus' --> 'Jia Kang'를 'Marcus'가 덮어버려서 Update!
- List에는 다양한 Data type을 넣을 수 있지만 비추.. --> 나중에 어느 항목이 뭐였는지 까먹음
그래서 보통 List에는 같은 type의 data를 넣는다!
List_test
List_test = [1,'Marcus',True,0.5959,'문자열']
List_test
[1, 'Marcus', True, 0.5959, '문자열']
List of Lists
- List 안에 List를 또 집어 넣을 수도 있다. 이 때 Element를 Call 하는 법? 아래와 같다.
2차원이라고 생각하면 편하지요~
people = [['180','Park'],['159','Han']]
print(people[0])
print(people[1])
print(people[0][0])
print(people[1][1])
['180', 'Park']
['159', 'Han']
180
Han
Operations on Lists
- List를 계속 만지작거리다보면 자연스럽게 익숙해지는 여러 Operation들!
- 구태여 외울필요도 없다. 무조건 익혀질
test = [1,2,-1,3]
print(len(test))
print(max(test))
print(min(test))
print(sum(test))
print(sorted(test)) # 오름차순 정렬
print(test+[5,6,7])
print(test*3)
del test[0] # List에서 0번째 Element 삭제
print(test)
4
3
-1
5
[-1, 1, 2, 3]
[1, 2, -1, 3, 5, 6, 7]
[1, 2, -1, 3, 1, 2, -1, 3, 1, 2, -1, 3]
[2, -1, 3]
- sorted에서 정렬을 내림차순으로 하고 싶다면?
(주피터노트북은 sorted치고 ALT+TAB+TAB하면 궁금할법한 사항들의 해결법이 어지간하면 제공된다)
sorted(test,reverse=True)
[3, 2, -1]
또 다른 List Methods
- 알아두면 유용쓰
people = ['Kim','Kim','Park','Lee']
people.count('Kim')
2
#########################
people = ['Kim','Kim','Park','Lee']
people.append('Han') # 맨 뒤에다가 Element를 추가한다
people
['Kim', 'Kim', 'Park', 'Lee', 'Han']
#########################
people = ['Kim','Kim','Park','Lee']
people.clear() #싹 제거!
people
[]
#########################
people = ['Kim','Kim','Park','Lee']
people.index('Lee') #요놈의 Index는 몇 번째인지
3
#########################
people = ['Kim','Kim','Park','Lee']
people.insert(2,'Han') # 2번째에 Han을 낑겨넣다
people
['Kim', 'Kim', 'Han', 'Park', 'Lee']
#########################
people = ['Kim','Kim','Park','Lee']
people.pop() # 맨 뒤에꺼 빼내기
print(people)
people.pop(0) # 0 번 째 지정해서 빼내는군
print(people)
['Kim', 'Kim', 'Park']
['Kim', 'Park']
#########################
people = ['Kim','Kim','Park','Lee']
people.remove('Park')
people
['Kim', 'Kim', 'Lee']
#########################
people = ['Kim','Kim','Park','Lee']
people.sort()
people
['Kim', 'Kim', 'Lee', 'Park']
#########################
people = ['Kim','Kim','Park','Lee']
people.reverse()
people
['Lee', 'Park', 'Kim', 'Kim']
Slicing
- a[ i : j ] : 실은 i 부터 j까지가 아니고 i 부터 j-1 까지의 Element들을 Cut! 해서 가져온다.
people = ['Kim','Park','Kim','Lee']
print(people[1:2])
print(people[1:3])
print(people[:]) # ALL
print(people[2:4])
print(people[2:3])
print(people[0:3])
print(people[:-1]) # 뒤에서 부터 하나 빼고 Slice
print(people[-3:-1])
['Park']
['Park', 'Kim']
['Kim', 'Park', 'Kim', 'Lee']
['Kim', 'Lee']
['Kim']
['Kim', 'Park', 'Kim']
['Kim', 'Park', 'Kim']
['Park', 'Kim']
Copy vs Alias
- Copy : people_copy = people[:] // Creates another list with the same elements!
Copy list를 수정해도 기존 리스트는 변화가 없다.
- Alias : people_alias = people // Another name referring to the same list.
alias를 수정하면 기존 리스트도 똑같이 변한다! 주의
for LOOP
- Form
for <variable> in <list> :
<block> #indentation 필수
- <list> 안에 있는 element들이 순서대로 variable로 들어가서 <block> 안에 있는 구문을 실행한다.
people = ['Kim','Park','Kim','Lee']
for name in people :
print(name)
Kim
Park
Kim
Lee
for LOOP with a Range of Numbers
- range function --> range(k) : 0부터 k-1까지 숫자가 있는 List를 만들어준다. (Element 총 k개)
- range(3,8) ? --> [3,4,5,6,7] --> 꼭 0부터 시작이 아닐 수도 있다.
- range(2,7,3) ? --> [2,5] --> 공차가 3인 등차수열
- for 문 예제 ex)
# 1부터 101까지 다 더하는 코드 using for문
total = 0
for i in range(0,101):
total += i
print(total)
5050
# List의 각 Element들을 2배 하는 코드 using for문
test = [1,2,3,4,5]
for i in range(len(test)):
test[i]=test[i]*2 #index로 접근을 해야 List값이 변한다!!
test
[2, 4, 6, 8, 10]
- for문 안에서 index로 접근을 안 하고 local variable로 접근하면 원래 list element가 바뀌지 않는다. local variable은 for문이 끝나면 사라지기 때문!( for num in test 이런식으로 접근하면 안 된다는 것 )
- for문과 if문 합친 예제 --> 사람이름과 번호 순서대로 출력해보기
1 st, 2 nd 이렇게 띄어져있는 걸 1st, 2nd로 붙쳐버리기 위해 i+1을 string으로 변환후 +로 더했다.
name = ['A','B','C','D','E']
num = [123,456,789,101112,131415]
for i in range(len(name)):
if i==0:
print(str(i+1)+"st is",name[i],'with num',num[i])
elif i==1:
print(str(i+1)+"nd is",name[i],'with num',num[i])
elif i==2:
print(str(i+1)+"rd is",name[i],'with num',num[i])
else:
print(str(i+1)+"th is",name[i],'with num',num[i])
1st is A with num 123
2nd is B with num 456
3rd is C with num 789
4th is D with num 101112
5th is E with num 131415
While Loop
- Form : Condition이 만족한다면 계속 block을 실행하라
while <condition>:
<block> # indentation 필수
- ex)
i=0
while i<5:
print(i)
i=i+1
0
1
2
3
4
- 위에서 Condition Variable이라는 i 값을 잘 조정해주지 않으면 while루프는 무한히 돈다!
- 무한루프에 빠졌다면 ? --> CTRL + C 로 Terminal 강제종료 가능
- While문 ex) end를 입력할 때까지 계속 input을 받는 코드 ㅎㅎ
test = 0
while test!='end':
test=input('그만하려면 end입력:')
if test=='aa':
print(test)
else:
print('다시~')
그만하려면 end입력:bb
다시~
그만하려면 end입력:cc
다시~
그만하려면 end입력:aa
aa
그만하려면 end입력:end
다시~
Loop Control : Break
- Break를 만나면 loop를 바로 나간다. (가장 가까운 for문 or while문 중단) ex)
for i in range(5):
print(i)
if i==3:break
0
1
2
3 # 3에서 break
Loop Control : Continue
- 현재 iteration만 중단하고 다시 LOOP를 돌려라
for i in range(6):
if i==3:
continue
elif i==5:
break
print(i)
0
1
2 # 3에서 Continue로 SKIP
4 # 5에서 Break
- Break / Continue는 어지간하면 코드 짜는데 추천하진 않는다. 여러 가지 위험부담이 있기에.. 최대한 For문으로 코드를 잘 짜고! While문을 피치 못하게 사용할 시 논리적으로 While문 잘 끝날 수 있게 조정하즈아!
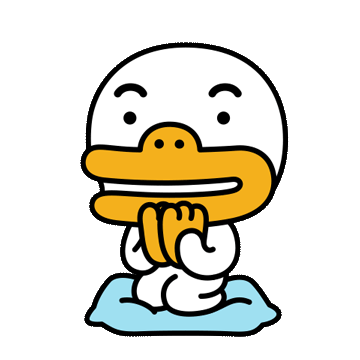
'SW 만학도 > Python' 카테고리의 다른 글
7. File I/O in Python (1) | 2024.03.15 |
---|---|
6. Sets, Tuples, and Dictionaries (2) | 2024.03.15 |
4. Modules and Classes in Python (0) | 2024.03.13 |
3. String and Control in Python (0) | 2024.03.13 |
2. Python Functions (2) | 2024.03.12 |