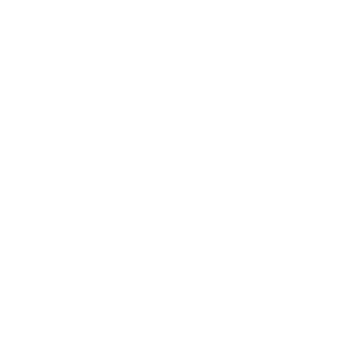
많고 많은 데이터 타입중에서 사실 우리 인간에게 가장 중요한 것은 문자, String Type이다.
돌풍을 몰고 온 Chat GPT도 인간의 언어, 자연어처리의 일종이니 말이다.
미래 먹거리! String type을 한 번 파헤쳐보자.
String Type
- Python은 int/float으로 Numeric Value를 표현한다.
- String(str) type으로 Text Value를 표현한다.
- Python은 문자열을 ' ' or " " 로 구분한다. ex) 'Gaza!!', "Lovey Dovey" (작은/큰따옴표 혼용 X)
- 25 vs "25" ? => 숫자 vs 문자
Built-in Operation with Strings
- len(str) : 띄어쓰기 포함, str의 길이를 반환 --> number of characters
- str1 + str2 : 숫자 + 문자는 X 이지만 문자 + 문자는 O --> 'How' + ' ' + 'do'+'?' => How do?
- str1*2 = str1str1 반복시키기 가능
- Type 바꾸기 : int('1') --> 1 , float('123.456') --> 123.456 , str(123) -> '123'
cf) int('가자') --> ERROR! 숫자만 int or float type으로 바꿔준다.
name = 'Marcus'
name + ' is a student'
'Marcus is a student'
Special Characters in Strings
- 문장 중간에 작은 따옴표(')가 있을 때? --> 큰 따옴표로 둘러싸라
test = "I'm a student"
test
"I'm a student"
- 문장 중간에 작은 따옴표 & 큰 따옴표 모두 있을 때? --> 역슬레시(\-->키보드에 원화표시) 사용
test2 = 'I said \"I\'m a student\"'
print(test2)
I said "I'm a student"
- 특수 케이스들
\' : Single quote
\" : Double quote
\\ : Backslash
\t : Tab
\n : Newl ine
Printing
- 파이썬 코딩 중간중간에 디버깅을 위해 꼭 필요한 함수(STEP별로 결과 값이 제대로 나오는 지 확인용)
- 위에서 배운 \t, \n 활용
print("A\tIs\nan\talphabet")
A Is
an alphabet
Getting an Input from Keyborad
- Printing과 반대되는 개념, User로부터 값을 받는다.
- ex)
a = input() #User가 123 타이핑함
123
print(a)
123
name = input("Plase type you name:") #User가 Marcus 타이핑!
Plase type you name:Marcus
print(name)
Marcus
Control in Python --> Boolean Type(True or False)
- Control flow statement using True or False
- Boolean operators : not, and, or
- ex) True and False = False , True or False = True (and는 둘 다 T 일 때만 T, or는 하나만 T여도 T)
- 계산 우선순위 : 1st not , 2nd and(곱셈) , 3rd or(덧셈)
- 헷갈리면 괄호를 써서 순서를 명시하자
print(True and False)
print(True or False)
print(not True or True and False)
print(not True or True and True)
print(not True and True or False)
False
True
False
True
False
Relational Operatiors
- 비교연산자
- ex) >,<,<=,>=,==(equal 주의! =:ASSIGNMENT ),!=(not equal)
print(False or 7+3>5 and 3==1)
print(False or 7+3>5 and 3!=1)
False
True
Short - Circuit evaluation
- 컴퓨터는 뒤 계산이 필요 없이 결과가 나오는 경우엔 효율적으로 뒷계산을 생략해버린다
- ex)
ASCII Code and Comparing Strings
- 문자열끼리 서로 크기비교를 하는 법
- 아스키코드 0~255 숫자에 대응되는 문자를 나열한 표.
A String inside Another String
- in Operator
- String에서 포함여부 확인하
'a' in 'I am a boy'
True
'c' in 'I am a boy'
False
------------------------
'' in 'I am a boy' # 공백은 모든 값에 포함이 됨(in 쓰면 무조건 True)
True
Control Statesment! IF ( 코딩의 꽃 )
- if statement로 프로그램을 실행시킬 지 여부를 결정할 수 있다. 기본틀은 아래와 같음
if <boolean_condition>: #Condition이 True일 때 밑에 블럭 실행
<block> #Indentation 필수
- ex) if(시작) / elif * n ( 계속 조건 추가 ) / else(마지막 나머지 조건 몰빵)
time=input('What time is it now?(HH:WW):')
What time is it now?(HH:WW):12:45
if time < "12:00":
print('Before lunch')
elif time <= "13:00":
print('It is lunch time!')
else:
print('After lunch')
It is lunch time!
------------------------------------
time=input('What time is it now?(HH:WW):')
What time is it now?(HH:WW):13:10
if time < "12:00":
print('Before lunch')
elif time <= "13:00":
print('It is lunch time!')
else:
print('After lunch')
After lunch
- Indentation만 있으면 IF안에 또 IF문을 만들 수도 있다.
- ex)
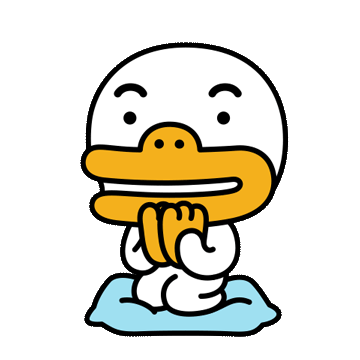
'SW 만학도 > Python' 카테고리의 다른 글
6. Sets, Tuples, and Dictionaries (2) | 2024.03.15 |
---|---|
5. Lists and Loops in Python (0) | 2024.03.14 |
4. Modules and Classes in Python (0) | 2024.03.13 |
2. Python Functions (2) | 2024.03.12 |
1. Hello Python (1) | 2024.03.12 |