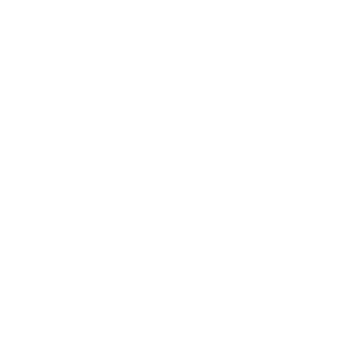
min, max 요런 거 말고 제대로 파이썬 함수를 만들어 보자!
Define Own Fucntion
- ex1) convert_to_fahrenheit(10)
- We want to get 50(Fahrenheit = Celsius * 9/5 + 32)
def convert_to_fahrenheit(celsius):
return celsius * 9/5 + 32
convert_to_fahrenheit(10)
50.0
위와 같은 형식으로 함수 선언
- 함수명을 모두가 이해할 수 있도록 지정 必
- Indentation 必, 몇 칸 띄는 지 정해지진 않았지만 몇 칸 이든 Consistent! 하게 공백유지
- ex2) 이번엔 반대로 Fahren -> Celsius로 코딩해보자
def convert_to_celsius(fahrenheit):
return (fahrenheit-32)*5/9
convert_to_celsius(50)
10.0
General Rule of Making Function :
def <function_name>(<parameters>):
<function body>
- Parameter를 여러 개 넣고 싶을 땐 콤마로 이어주면 된다.
- ex) def sum(a,b,c) : return a+b+c
- Funtion call? : return <expression> 부분이 계산되어 function call의 결과값으로 반환
Local Variables
- Function 안 에서 만들어진 변수들
- Funtion 밖에선 쓰일 수 없다. Funtion 안에서만 쓰이고 없어진다.(Temporarily store intermediate results)
- Parameter도 Local Variable이다.
Namespace
- Function이 실행 중일 땐 current namespace는 지금 이 Function이다! 함수 밖에 선언된 Global variable말고 함수 안에 선언된 Local Variable만 신경쓰자.(Global Variable과 Local Variable의 이름이 같을 때 주의하라는 뜻! 아래 참고해보자)
a=10
def test(b):
return b+a
test(3)
13
-----------------------
a=10
def test(b):
a=5
return b+a
test(3)
8
Funtion call overview :
- 1. Evaluate the argument left to right ( 함수 파라미터 대입시 왼->오른쪽 순으로 순서에 맞게 입력 )
- 2. Create a namespace to hold the function call's local variables, including the parameters
- 3. Pass the resulting values into the function by assigning them to the parameters
- 4. Execute the function body then return statement.
- ex)
def doubling(x):
return x*2
x=5
x=doubling(x+5)
print(x)
20
Function 만드는 꿀팁 내지는 Guidelines
- Must have a plan(a.k.a. algorithm) in advance
- What is plan? : 함수의 기능이 뭘까, 그 기능을 나타내는 함수명, 인자(Parameter)를 뭘 받을까? 그 걸로 Body 어떻게 계산할까? 어떤 값을 Return하면 될까? 함수 다 만들고 QA를 위해 Test도 진행해보자!
- ex) 두 날짜의 차이를 계산하는 function을 만들라
# int인 날짜 2개를 받았을 때 그 두 날짜의 차이를 계산하는 Function이다.
def days_difference(day1:int,day2:int) -> int:
return abs(day1-day2)
days_difference(3,5)
2
위와 같이 Function을 만들 때, 선택사항이지만 Parameter의 data type, return값의 data type도 친절히 써주고
주석처리를 해서 함수에 대한 설명도 친절하게 달아주자~~
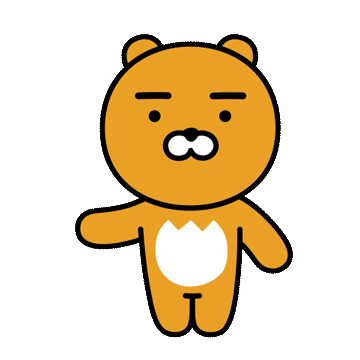
'SW 만학도 > Python' 카테고리의 다른 글
6. Sets, Tuples, and Dictionaries (2) | 2024.03.15 |
---|---|
5. Lists and Loops in Python (0) | 2024.03.14 |
4. Modules and Classes in Python (0) | 2024.03.13 |
3. String and Control in Python (0) | 2024.03.13 |
1. Hello Python (1) | 2024.03.12 |