You are given a 0-indexed integer array nums of length n.
nums contains a valid split at index i if the following are true:
- The sum of the first i + 1 elements is greater than or equal to the sum of the last n - i - 1 elements.
- There is at least one element to the right of i. That is, 0 <= i < n - 1.
Return the number of valid splits in nums.
Example 1:
Input: nums = [10,4,-8,7]
Output: 2
Explanation:
There are three ways of splitting nums into two non-empty parts:
- Split nums at index 0. Then, the first part is [10], and its sum is 10. The second part is [4,-8,7], and its sum is 3. Since 10 >= 3, i = 0 is a valid split.
- Split nums at index 1. Then, the first part is [10,4], and its sum is 14. The second part is [-8,7], and its sum is -1. Since 14 >= -1, i = 1 is a valid split.
- Split nums at index 2. Then, the first part is [10,4,-8], and its sum is 6. The second part is [7], and its sum is 7. Since 6 < 7, i = 2 is not a valid split.
Thus, the number of valid splits in nums is 2.
Example 2:
Input: nums = [2,3,1,0]
Output: 2
Explanation:
There are two valid splits in nums:
- Split nums at index 1. Then, the first part is [2,3], and its sum is 5. The second part is [1,0], and its sum is 1. Since 5 >= 1, i = 1 is a valid split.
- Split nums at index 2. Then, the first part is [2,3,1], and its sum is 6. The second part is [0], and its sum is 0. Since 6 >= 0, i = 2 is a valid split.
Constraints:
- 2 <= nums.length <= 105
- -105 <= nums[i] <= 105
지난 번과 비슷한 prefix sum 문제이다.
문제 이해는 완료했고, 그리 어려워 보이진 않다만.. 역시 indexing을 어떻게 detail하게 잘 하냐가 관건일듯 싶다.
1. Python
다행이 나는 그래도 한 번 배우면 어느 정도까지는 풀이법을 기억할 줄 아는 Memory를 가지고 있었다..
최악은 아니라는 뜻 ㅎㅎ
쉽게 해결 完!
class Solution:
def waysToSplitArray(self, nums: List[int]) -> int:
n = len(nums)
prefix = [0]*n
prefix[0] = nums[0]
# O(N)
for i in range(1,n):
prefix[i] = prefix[i-1] + nums[i]
largest = prefix[-1]
ans = 0
# O(N)
for j in range(n-1):
# if prefix[j] >= largest - prefix[j] : ans += 1
if prefix[j]*2 >= largest : ans += 1
return ans
2. C / C++로도 함 해볼까나
int waysToSplitArray(int* nums, int numsSize) {
long long prefix[numsSize];
prefix[0] = nums[0];
for(int i=1;i<numsSize;i++){
prefix[i] = prefix[i-1] + nums[i];
}
long long largest = prefix[numsSize-1];
int ans = 0;
for(int j=0;j<numsSize-1;j++){
if(prefix[j]*2 >= largest) ans++;
}
return ans;
}
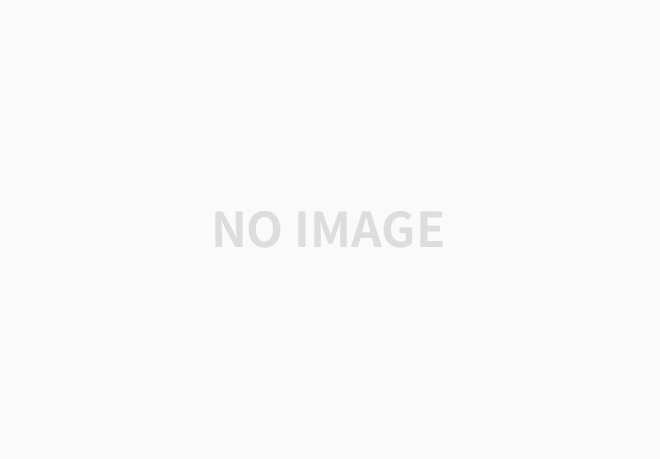
C는 test case에서 overflow 발생하는 게 있어서 long long 변수를 사용했다;;
C++도 마찬가지..
class Solution {
public:
int waysToSplitArray(vector<int>& nums) {
int n = nums.size();
vector<long long> prefix(n,0);
prefix[0] = nums[0];
for(int i=1;i<n;i++){
prefix[i] = prefix[i-1] + nums[i];
}
int ans = 0;
long long largest = prefix[n-1];
for(int j=0;j<n-1;j++){
if(prefix[j]*2 >= largest) ans++;
}
return ans;
}
};