2-1. Operators / Memory in C
https://eglife.tistory.com/32 2. Variables in C 아무리 봐도 불친절한 C.. 파이썬을 배우다가 와서 그런가 불편한 게 이만 저만이 아니다. 그래도 파이썬코드보다 처리속도도 빠르고, 디버깅에 유용하다니
eglife.tistory.com
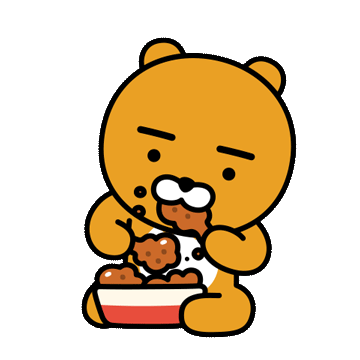
C에 대해서 좀 더 톺아보는 시간!
C는 Python과 달리 Vairable의 Data type을 뭘로 쓸 지 Compiler에게 미리 다 알려줘야 한다.
C에서 IF / ELSE 구문이 어떻게 작용되는지 체크
IF Statement
- IF문 안에 Mulit Statement가 아니라면 굳이 중괄호는 쓸 필요가 없지만, 그래도 혹시나 발생할 수 있는 Error를 방지하고자 if문엔 어지간하면 중괄호를 쓸 것
#include <stdio.h>
// Month를 input으로 받고 그 달이 며칠로 이루어져 있는지 리턴하는 함수
int main(void)
{
int month;
printf("Enter the month: ");
scanf("%d",&month);
if(month==4||month==6||month==9||
month==11){
printf("Month has 30days\n");
}
else if(month==1||month==3||month==5||
month==7||month==8||month==10||month==12)
{ printf("Month has 31days\n");
}
else if(month==2)
{
printf("28days");
}
else
{ printf("I dont know that month\n") ;
}
return 0;
}
- else는 가장 가까운 IF와 연관지어진다. Python에선 같은 Indentation이면 되는데, C는 구분x
Switch Statement
- If / Else 구문과 비슷하다. 다만 "case"라는 것을 사용해서 구분짓는다. 다만 꼭 "break;"를 덧붙혀 줘야 한다.
- Case는 Starting Point이지 End Point는 아니어서, break;가 없다면 코드가 아래 구문까지 쭈욱 실행된다.
int main(void)
{ char input;
printf("ender an Alphabet A-C\n");
scanf("%s",&input);
switch(input){
case 'A':
printf("its A\n");
break;
case 'B':
printf("its B\n");
break;
case 'C':
printf("its C\n");
break;
default:
printf("Out of Range\n");
}
return 0;
}
- case 'A' 에 break가 없고, input으로 A를 입력하면 Output은 아래와 같이 나온다. (break 없으면 코드는 지속됨)
its A
its B
- Default 값은 어느 case에도 걸리지 않을 때 실행되는 구문이다.
Iteration in C
1) While Statement
int main(void)
{
int x = 0;
while(x<5)
{
printf("%d",x);
x++;
}
// 01234
return 0;
}
2) Do - While Statement
- Loop Body를 먼저 일단 실행하고, 그 다음 계속 할지 여부를 While로 결정을 한다.
int main(void)
{
int x = 0;
do{
printf("%d ",x);
++x; // 참고: x++는 일단 x를 왼쪽 변수에 대입하고 그 다음 x=x+1 한다.
}while(x<5); //while 문 뒤에 세미콜론 필수!!
// 0 1 2 3 4
return 0;
}
int main(void)
{
int x = 0;
do{
printf("%d ",x);
++x; // 참고: x++는 일단 x를 왼쪽 변수에 대입하고 그 다음 x=x+1 한다.
}while(x<-1); //while 문 뒤에 세미콜론 필수!!
// 0
return 0;
}
- Do / While문은 일단 Body문을 실행하기 때문에 while에 걸리지 않아도 '0'은 출력된다.
For Statement
- while문 보단 for문이 좀 더 안전하긴 하다.
int main(void)
{
int x = 100;
for(x=0;x<5;x++)
{
printf("%d ",x);
}
// 0 1 2 3 4
return 0;
}
- 만약에 iterator x 를 사전에 선언하지 않고 for문에 바로 쓰고 싶다면?
int main(void)
{
int x = 100;
for(x=0;x<5;x++)
{
printf("%d ",x);
}
printf("%d\n",x); //x가 처음 100에서 for문 끝나고 5로 자동 업데이트 되어버린다. 의도치 않은 결과를 초래할 수도 있음
for(int i=4;i>=0;--i){
printf("%d ",i);
} // iterator를 for문 안에 써주려면 int i 이런식으로 해야 함
// 4 3 2 1 0
return 0;
}
int main(void)
{
for (int x=1;x<10;x++){
for(int y=1;y<10;y++){
printf("%d\t",x*y);
}
printf("\n");
} // 구구단
// 1 2 3 4 5 6 7 8 9
// 2 4 6 8 10 12 14 16 18
// 3 6 9 12 15 18 21 24 27
// 4 8 12 16 20 24 28 32 36
// 5 10 15 20 25 30 35 40 45
// 6 12 18 24 30 36 42 48 54
// 7 14 21 28 35 42 49 56 63
// 8 16 24 32 40 48 56 64 72
// 9 18 27 36 45 54 63 72 81
return 0;
}
Break and Continue Statements
- Break : LOOP 시마이! , Continue : 이번 turn만 SKIP!
int main(void)
{
for(int x=0;x<10;x++){
if(x==5){
break;
}
printf("%d\t",x);
}
printf("\n");
for(int x=0;x<10;x++){
if(x==5){
continue;
}
printf("%d\t",x);
}
// 0 1 2 3 4 --> 5에서 끝
// 0 1 2 3 4 6 7 8 9 --> 5만 스킵
return 0;
Summary Exercise
- 계산기 만들어보자
int main(void)
{
float x,y;
char oper;
printf("1st num?\n");
scanf("%f",&x);
printf("operation type (+ or - or /) ?\n");
scanf("\n%c",&oper); // 이렇게 해야 oper 받는게 안 씹힌다..
printf("2nd num?\n");
scanf("%f",&y);
float result;
switch(oper){
case '+':
result= x+y;
break;
case '-':
result= x-y;
break;
case '/':
if(y==0){
printf("Error\n");
return 0;
}
else{
result = x/y;
}
break;
default:
printf("Error!\n");
return 0;
}
printf("result : %0.3f \n",result);
return 0;
}
요기까지는 할만한 C의 세계~ ㅋㅋㅋ
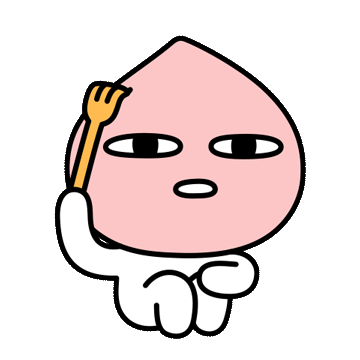
'SW 만학도 > C' 카테고리의 다른 글
5. Pointer - Motivation in C (1) | 2024.03.26 |
---|---|
4. Function ic C - Grammer (1) | 2024.03.25 |
2-1. Operators / Memory in C (0) | 2024.03.20 |
2. Variables in C (0) | 2024.03.20 |
1. Hello C !!! Sorry for late.. (2) | 2024.03.19 |